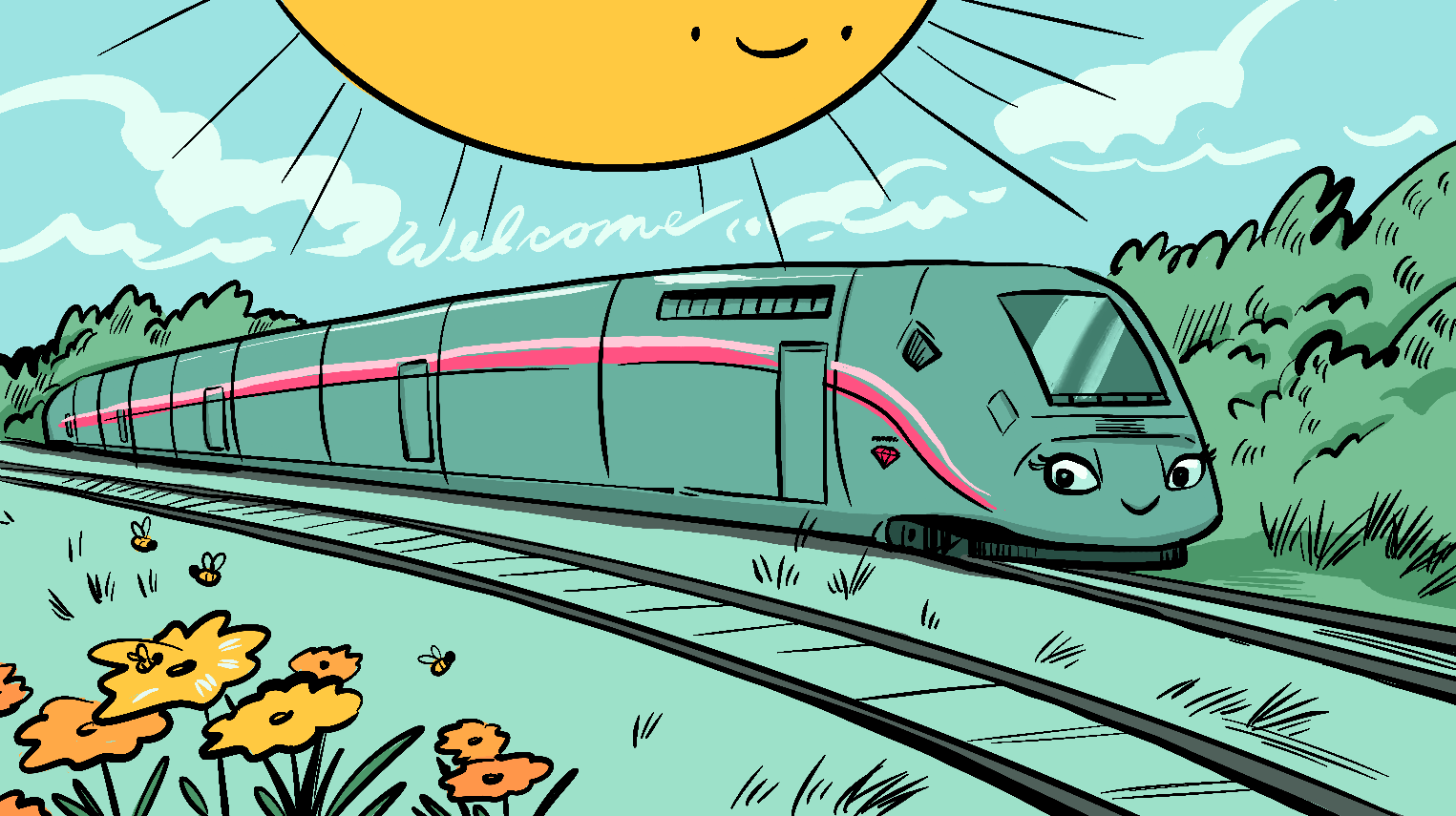
Rails has been around since early 2004. Back then, the choices of building web applications was either working with a bunch of spaghetti code that was SFTP'ed up to a server or work with an enterprise monstrosity like Enterprise Java Beans. Rails showed us that web development could be fun and sane with built-in features like environments, database migrations, and a Model-View-Controller approach to building web applications.
Let’s do some math. The year at the time of this writing is 2023. Rails was created on August of 2004. Rails is over 18 years old! And to this day, after all this time, you’ll find passionate long-time Rails developers who swear by the framework as being the most productive for building and shipping applications, but many have taken for granted what it’s like to get started in Rails today and the plethora of other frameworks that have caught up.
Why should you consider Rails?
Rails (and Ruby) cares a lot about developer happiness and productivity. It optimizes for the great feeling of, “wow, I got a lot done today”. You start by building a web application, which can be integrated into an Android & iOS application (more on that later).
Reading the Rails Doctrine is a great place to get your bearings to put into context what the Rails community aspires to be. Despite those aspirations, you’ll sometimes find yourself in very disorienting places in Rails, like the asset pipeline. Overall though, Rails is quite an enjoyable developer experience.
Getting Started
If you’re getting started for the very first time with Rails you’ll want to have these resources open and ready in your browser as you build a Rails application.
Rails Guides
Rails Guides are a great place to start reading about the basics of building a Rails application. Even when you’ve moved beyond the basics, you’ll still find yourself landing on guides to understand how a specific Rails library works.
Rails API
When your journey moves past tutorials, you’ll find yourself referring to the API docs to see how specific classes or methods work in Rails. Its a reference that you’ll want to keep handy.
Ruby Docs
Just like the Rails API, there’s loads of stuff that you’ll be using from the Ruby language and its standard runtime. Sometimes it’s hard to know what’s in Ruby vs what’s in Rails, especially because of a huge library in Rails that extends the Ruby language called Active Support.
Chat GPT
As large language models, like OpenAI’s Chat GPT-4 and Google’s Bard, become ever more capable, they also becoming better tools to help answer questions from people who are starting out in any new programming language. GPT-4 is a great resource for getting help, but be warned that its perfectly happy giving answers that seem plausible, but are either completely wrong, convoluted, or not a best practice.
Stuff you’ll inevitably bump into with Rails
It might not say it in the beginners guides, but most people who ship Rails applications are using these technologies in their stack.
Postgres
rails new
defaults to sqlite, which is a really great database, but when it’s time to deploy to production, most people choose Postgres as their database.
Redis
I joke, “it really should be called Rails on Redis”, which is kind of true. Redis can be used in Rails for caches, background job processing, and WebSockets, ActionCable, and Hotwired.
Redis is really a Swiss Army knife. It’s great for caches because it’s really fast to read and write data to Redis. It also ships with pub/sub features that make it suitable for use by background workers and pushing notifications to browsers via WebSockets.
Sidekiq
Web requests should be fast, but sometimes your application needs to do something that takes a few minutes, like change the resolution of a large video file. The last thing you want in a web application is have a web server take a few minutes to fulfill a request—doing so would probably bring your web application to a crawl as requests stack up in a queue waiting for the server to respond.
The solution in Rails is to offload this request to a background worker, which frees up the web server to continue taking requests from other clients.
Sidekiq is the most popular background worker framework. You can’t go wrong using it since its battle tested in production by many large Rails applications.
Hotwire
Hotwire is part of Rails, but if you landed on their website you’d only see Rails mentioned as a footnote. Inside Rails, you’ll see these referred to as Turbo and Stimulus.
Hotwire was created by the same people who created Rails in an effort to drastically reduce the amount of JavaScript needed to be written and deployed to the client. This approach to building low latency interactive web applications has surged in popularity over the past few years to combat the increasing complexity and frustrations Rails developers experienced when building JS web applications.
The framework is included by default when you create a new Rails application.
Ruby Gems & Bundler
At the root of your Rails application you’ll see a Gemfile
. The entries in that file point to packages maintained by a community of Ruby developers that are hosted on https://rubygems.org.
Screencasts
When you’re tired of reading documentation and simply want to sit on the couch and watch Rails, there’s a few notable screencasts worth watching.
GoRails
GoRails covers a wide variety of Rails & Ruby topics over a huge library of videos. Its really a great place for beginners who prefer to be lead step-by-step through a problem to learn.
RailsCasts
A classic for those who got started a decade ago and still surprisingly relevant today. The Pro videos were recently made free by the author because the videos are dated.
Extending Rails
As you start adding more features to your application, you will begin looking at plugins that add features to Rails apps like authentication, payment, and more. Fortunately there’s some really great resources out there to help you figure out which gem is most suitable for your project.
The Ruby Toolbox
When I do research to see what RubyGem I need to install in Rails to solve a problem, the first place I look as https://www.ruby-toolbox.com to see what other similar libraries exist. This helps me make a more informed decision about which library I should close.
Awesome Ruby
Awesome Ruby is a more brief list of the popular RubyGem’s that the Ruby and Rails community use in their applications.
Newsletters
You don’t need to subscribe to all of them, but its worth picking one to read at least once a week so you can keep track of all the new stuff and changes that comes to Rails.
Ruby Weekly
Includes general news & updates about Ruby & Rails, links to new tutorials & articles, and a section on code & tools that are new or have been updated.
Short Ruby News
A summary of Tweets & Toots from people working on interesting problems within the Rails and Ruby community.
This Week in Rails
A weekly newsletter from the Rails team that covers changes and new features that land in Rails. This news letter is more narrowly focused on changes to the Rails framework, so you’d miss out on a lot of other news about Ruby Gems, plugins, and interesting community tutorials if you only subscribe to this.
Mobile Apps
If you get to a point where you want to ship a mobile application for Rails, there’s a whole stack for that. The team behind Rails has also released libraries that make it possible to easier integrate a Rails application with mobile apps.
Turbo iOS & Android
The team behind Rails ships a library that makes basic integration with Rails possible. There’s still a lot of gaps in the framework that you’ll need to fill in, like authentication.
The Turbo Native Guy
Joe Masilotti has been building Turbo iOS applications for years and writes about them on his website. A lot of his content gives some pretty great answers to the gaps left open in the Turbo Native frameworks. Joe has even released his own starter kit called Turbo Navigator that fills in a lot of the gaps left open in the Turbo iOS project.
Sharp edges
Like anything, Rails has sharp edges that are worth knowing about when you start using it.
JavaScript, CSS, and image assets
I’ve written about the asset pipeline being confusing. The default importmaps pipeline is reasonable for somebody shipping a new app, but the moment you need to use a JavaScript package the requires compilation — or a node package’s CSS files, images, or other assets, you’ll find that the path forward can become overwhelming.
Deployments
There’s no one way to deploy a Rails application, which is a decision that a person new to Rails has to make. Fortunately the upcoming Rails 7.1 release will include a way to generate Dockerfiles, which provide a standard configuration of the server you’ll deploy to production.
Better yet, the dockerfile-rails
gem can be added to any Rails project to automatically generate Dockerfiles that can be deployed to host like Fly.io that accept Dockerfiles. Here’s how you can quickly generate a Dockerfile in your Rails app and deploy to production.
$ bundle add dockerfile-rails
$ bin/rails g dockerfile
$ brew add flyctl
$ flyctl launch
$ fly open
If that all goes well you’ll see a running Rails app.
Hotwire
Hotwire makes it possible to build responsive & realtime web applications without a ton of JavaScript. The documentation at https://hotwired.dev/ was written more for the front-end so it could be agnostic to Rails, which makes it harder to follow at times.
Rails ships with some helpers, called Turbo Frames and Turbo Stream, that are mostly documented in the Github repo at https://github.com/hotwired/turbo-rails, but not yet in the Rails guides.
“Too much magic”
If you fired up a Rails console via bin/console rails
you’d find the following commands work as you’d expect
irb(main):001:0> 24.megabytes
=> 25165824
irb(main):002:0> 1.month.from_now
=> Fri, 07 Apr 2023 00:45:35.762342000 UTC +00:00
But if you ran the same thing in “Regular Ruby” via irb
none of them would work. That’s because Rails adds methods to core Ruby classes that gives them super powers called Active Support. You’ll find yourself switching between these docs and Ruby’s docs.
This is the tip of the iceberg
There’s so many amazing people in the Ruby and Rails community shipping incredible stuff. What’s really great about the Rails community is how many resources are available for everybody using Rails including folks just starting out all the way up to the most Sr Rails engineers.
The best thing you can do to get started is pick a problem you’d like to solve, then build a solution for it in Rails and see how far you can get with the resources above.